How do I debug my lightning component?
General Tools and settings:
- use Chrome + built in Dev Tools + Lightning Component Inspector
- be sure debug mode is enabled in your org (Setup: Lightning Components)
- be sure caching is disabled in your org (Setup: Session Settings -> uncheck "Enable secure and persistent browser caching to improve performance"
Techniques for most common use cases (be sure that Dev Tools in Chrome are open while testing):
- check certain JavaScript code is called at all:
useconsole.log()
,console.debug()
,console.warn()
,console.error()
(different color visualizations) -> can output a variable value as well - check for invalid values only:
rather always log to the console you can doconsole.assert(myVar != 0)
to only show an error when assertion fails - evaluate variables / context on a certain JavaScript code:
usedebugger;
(this will create a breakpoint in code execution) -> now use the console from Dev Tools while breakpoint is active.
You can write JavaScript to test value of variables, do function calls and check return types, overwrite JavaScript values, and so on. This is super powerful also developing your code - output the value of a more complex Object:
while code execution is paused on a breakpoint (see above) usecopy(JSON.stringify(myObjectToEvaluate));
in the Dev Tool console - this will copy the value as JSON to your clipboard and you can process/inspect text based value - measure code execution time between two dedicated "anchors": use
console.time("identifier"); [code to execute] console.timeEnd("identifier");
- check why code can not be saved or application is not loading at all: use the Salesforce Lightning CLI to lint your JavaScript code (very useful to check against faulty structure like missing braces)
- investigate runtime exceptions origin:
sometimes runtime exceptions show up you don't know exactly where they come from. Use "Pause on Exception"-Button under the "Sources"-Tab in Dev Tools - inside the panel "Call Stack" check if you can identify classes from your code.
As code execution is paused you have the same behavior as a manual breakpoint: on every entry you are in the right JavaScript context so you can evaluate all values at that certain code execution time. Add some additional breakpoints (by clicking the line number in the Dev Tools) and you will always pause the code on certain area (like to check what values are before the exception raises or pause on code you can't write a debugger-statement by yourself) - mock data returned from you Apex controller: use the Lightning Components Inspector "Action" Tab and overwrite the result coming back from the server the next time
Way to Debug Salesforce Lightning Components
The Lightning Component framework is a UI framework for developing dynamic web apps for mobile and desktop devices. The framework is built on the open source Aura framework. It is used mostly for single page application(SPA).
After developing an application, if the developer needs to debug the lightning components we must follow the below steps.
Step 1: To enable debug mode for your organization (From Setup > Lightning components > Enable lightning debug mode). After enabling this option, it is easier to debug JavaScript code in your lightning components.
Step 2: To install Salesforce Lightning Inspector in your google chrome navigate to the https://chrome.google.com/webstore/detail/salesforce-lightning-insp/pcpmcffcomlcjgpcheokdfcjipanjdpc and click the ADD TO CHROME button.
Step 3: Now, browse to a lightning application in google chrome. You can see the Salesforce Lightning inspector adds a tab in your Chrome developer tools(F12). Also, you could see different subtabs to inspect different aspects.
Step 4: There are some tabs available, and it is listed below
- Component Tree Tab
- Performance Tab
- Transactions Tab
- Event Log Tab
- Actions Tab
- Storage Tab
Step 5: To debug your JavaScript code, for example while clicking the button, we want to check the functionality inside your method by using use “debugger” keyword. Simply add it wherever you want in your code.
Step 6: After placing the debugger inside your method, the inspector will stop running your code when it reaches to the “debugger” keyword.
Step 7: Then, we have an option to print log messages using JavaScript, and console.log (). In console tab, we will check the log messages of the Lightning components.
Salesforce: Debugging JavaScript
with 15 comments

Alert(). Old skool and not in a good way
I love JavaScript, and working within the Force.comPlatform I’m always finding new ways that it can be applied to business needs. I know this is the case for many developers out there but I’ve seen that their typical development process is:
- Develop the JS code
- Create bug
- Include calls to the alert() method to troubleshoot
- Iterate
alert() is bad for debugging
I’m sure this process sounds familiar to many of you, but do you know what, the alert() method is a very weak way of troubleshooting. I abandoned it years back because of poor features such as outputting object representations as ‘[object]’ or ‘[undefined]’ – which doesn’t give you a lot to go on.
Meet console.log()
Modern browsers have access to a library called ‘console‘ which offers a far richer experience, and without the annoying pop-ups. The console.log() method accepts any JavaScript type as a parameter and will always give you detailed and sensible output. In the images below I’ve passed the method a variable representing a Divand the log outputs the entire DOM from the Div down. You can even pass it jQuery objects and it’ll output all of that object’s associated data!
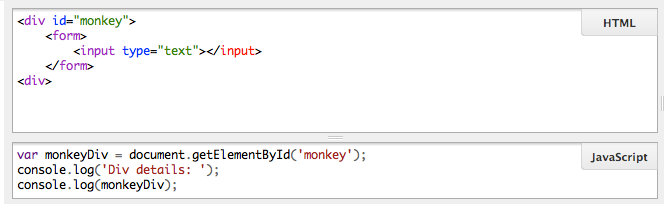
Console. Unobtrusive with richer output.

Now that's what I call a debugger!
So where does it output to? On the console tab of course. I almost always use Chrome these days and you’ll find the console tab if you open Chrome’s Developer Tools. It’s similarly placed within Firebug for Firefox.
For those who’d like to learn more about the console library here’s an excellent article. There you’ll see that the library has far more to offer than just the log() method.
Debugging Custom JavaScript Button
A neat tip to remember is that console.log() is available wherever JavaScript is used on the platform. That means you can even debug the JavaScript you use to access the AJAX API through Custom Buttons!